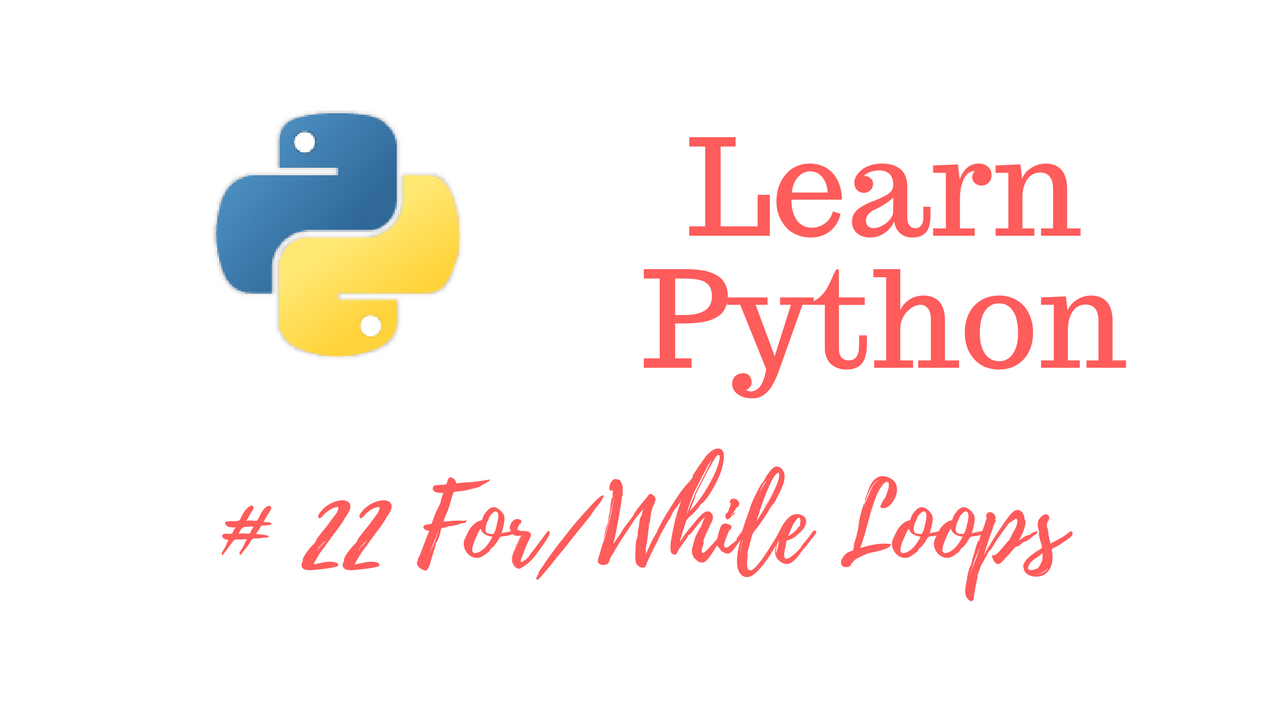
Get The Learn to Code Course Bundle!
https://josephdelgadillo.com/product/learn-to-code-course-bundle/
Enroll in The Complete Python Course on Udemy!
https://www.udemy.com/python-complete/?couponCode=PYTHONWP
In this tutorial we are going to cover the two loops types in Python. The first one is a for loop. A for loop will allow you to iterate over a list in Python. In other words, you can do something for each item in the list. So, let’s go ahead and create a list.
numbers = [1, 2, 3, 4, 5]
for item in numbers
print(item)
When we run this each number in our list will be printed out in the console. Let’s add names to our list instead of numbers.
names = ["Nick", "Someone", "Another Person"]
for item in names
print("This persons name is", item)
That is a for loop, and basically the second parameter is to access the list, and then the first parameter is what you want each item in the list to be called while inside it’s little block of code. Now we’re going to learn about a while loop.
run = True
current = 1
while run:
if current == 100:
run = False
else:
print(current)
current += 1
In this bit of code we are creating two variables, while, and then we write what we want to happen while the program is running. In this case, we are going to check to see if current = 100. If not, we are going to add 1 to the total, and we are starting from 1. Once the total hits 100 the program will stop running. We will be using loops quite a bit throughout this course, so make sure you’ve mastered this concept.
Web – https://josephdelgadillo.com/
Subscribe – https://goo.gl/tkaGgy
Follow for Updates – https://steemit.com/@jo3potato